API: Users
Jump to: List | Create | Show | Update | Delete | Get current user | Get avatar | Get authentication token
List users
GET /api/users
Return a list of all active users in the account (doesn’t return any deactivated users).
$ curl -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/users.json
[
{
"user": {
"week_start": null,
"id": 5538,
"last_name": "Freckle",
"permissions": "integration, time, invoicing, reports, tags,
expenses, projects, team, people, account, billing, import",
"login": "admin",
"time_format": "fraction",
"email": "apitestadmin@letsfreckle.com",
"first_name": "Lets"
}
},
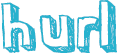
$ curl -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/users.xml
<?xml version="1.0" encoding="UTF-8"?>
<users type="array">
<user>
<email>apitestadmin@letsfreckle.com</email>
<first-name>Lets</first-name>
<id type="integer">5538</id>
<last-name>Freckle</last-name>
<login>admin</login>
<time-format>fraction</time-format>
<week-start nil="true"></week-start>
<permissions>integration, time, invoicing, reports, tags,
expenses, projects, team, people, account, billing, import</permissions>
</user>
<!-- ...more users... -->
</users>
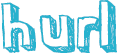
Response codes
Returns 200 OK
and an array of users in the response body.
Roles
All roles except the freelancer role can access this resource.
Show user
GET /api/users/<id>
Returns details about a specific user. This method works with active and deactivated users.
$ curl -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/users/5538.json
{
"user": {
"week_start": null,
"id": 5538,
"last_name": "Freckle",
"permissions": "integration, time, invoicing, reports, tags,
expenses, projects, team, people, account, billing, import",
"time_format": "fraction",
"email": "apitestadmin@letsfreckle.com",
"first_name": "Lets"
}
}
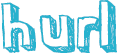
$ curl -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/users/5538.xml
<?xml version="1.0" encoding="UTF-8"?>
<user>
<email>apitestadmin@letsfreckle.com</email>
<first-name>Lets</first-name>
<id type="integer">5538</id>
<last-name>Freckle</last-name>
<time-format>fraction</time-format>
<week-start nil="true"></week-start>
<permissions>integration, time, invoicing, reports, tags,
expenses, projects, team, people, account, billing, import</permissions>
</user>
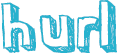
Response codes
Returns 200 OK
and a users in the response body.
401 Unauthorized
is returned if there’s no user with the given ID.
(This will be changed to 404 Not Found
in a future version of the API).
Roles
A freelancer can only see details about him- or herself. Other roles have access to all users in the account.
Get current user
GET /api/users/self
Returns details about the currently logged in user.
This method is otherwise identical with GET /api/users/<id>
.
Get a user’s avatar
GET /api/users/<id>/avatar
Returns URLs to a user’s avatar & thumbnail. If you build a client application, we strongly suggest caching these images.
$ curl -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/users/5538/avatar.json
{
"thumbnail": "http://apitest.letsfreckle.com/images/avatars/0000/0001/avatar_profile.jpg",
"id": 5538,
"avatar": "http://apitest.letsfreckle.com/images/avatars/0000/0001/avatar.jpg"
}
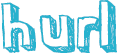
$ curl -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/users/5538/avatar.xml
<?xml version="1.0" encoding="UTF-8"?>
<user>
<thumbnail>http://apitest.letsfreckle.com/images/avatars/0000/0001/avatar_profile.jpg</thumbnail>
<avatar>http://apitest.letsfreckle.com/images/avatars/0000/0001/avatar.jpg</avatar>
<id type="integer">5538</id>
</user>
<?xml version="1.0" encoding="UTF-8"?>
<user>
<thumbnail></thumbnail>
<avatar></avatar>
<id type="integer">5538</id>
</user>
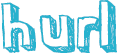
Response codes
Returns 200 OK
and the avatar URLs in the response body.
401 Unauthorized
is returned if there’s no user with the given ID.
(This will be changed to 404 Not Found
in a future version of the API).
Roles
The freelancer can only see the avatar for him- or herself. Other roles have access to all users in the account.
Get authentication token
GET /api/user/api_auth_token
The authentication token can be retrieved through the api_auth_token
resource.
3rd-party applications may prefer to ask their users for their email and password
instead of the authentication token because those are easier to remember.
We strongly recommend using this method only for interactive applications.
Note that this part of the API uses HTTP Basic Authentication instead of token authentication.
We plan to support OAuth authentication in a future API version.
$ curl https://test:testtest@apitest.letsfreckle.com/api/user/api_auth_token.json
{
"user": {
"api_auth_token": "lx3gi6pxdjtjn57afp8c2bv1me7g89j"
}
}
$ curl https://test:testtest@apitest.letsfreckle.com/api/user/api_auth_token.xml
<?xml version="1.0" encoding="UTF-8"?>
<user>
<api-auth-token>lx3gi6pxdjtjn57afp8c2bv1me7g89j</api-auth-token>
</user>
Response codes
If the HTTP Basic Auth email and password are correct, returns
200 OK
and the token information in the response body.
401 Unauthorized
is returned if authentication with
email and password.
Roles
Anyone can retrieve their authentication token using this resource.
Create user
POST /api/users
You can create new users, as long as your account limits are not reached. This method, as well as the update and delete methods, are intended to make it easier to synchronize users with your own systems. Note that you can’t set or change passwords.
Only the first_name
, last_name
and email
fields can be set.
Creating a user immediately sends them an invite email to the Freckle account.
Request example, expects a users.xml
file in the current directory:
$ curl -d @data/users.xml -H "Content-type: text/xml" -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j https://somaccount.letsfreckle.com/api/users.xml
Sample post body:
<?xml version="1.0" encoding="UTF-8"?>
<user>
<email>foobar@letsfreckle.com</user>
<first_name>foo</first_name>
<last_name>bar</last_name>
</user>
Response codes
201 Created
means that the user was successfully created.
The Location
header in the HTTP response contains the path to this
new user (in the API). This path contains the user ID if the newly
created user.
Roles
Only administrators and the account owner can use this API method.
Update user
PUT /api/users/<id>
The method updates a user’s name and email address.
Request example, expects a users.xml
file in the current directory:
$ curl -d @user.xml -X PUT -H "Content-type: text/xml" -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://somaccount.letsfreckle.com/api/user/ID.xml
Request body example:
<?xml version="1.0" encoding="UTF-8"?>
<user>
<email>foobar@letsfreckle.com</user>
<first_name>foo</first_name>
<last_name>bar</last_name>
</user>
Response codes
200 OK
is returned if the user was successfully updated.
422 Unprocessable Entity
is returned in case the API user
is not authorized to manage users in the account or if the given
data was invalid (for example, email address is a invalid format).
Roles
Only administrators and the account owner can use this API method.
Delete user
DELETE /api/users/<id>
Deactivates a user.
Users are never actually deleted, just deactivated. Currently, reactivation of a user is only available on the team page in the Freckle interface.
$ curl -X DELETE -H "X-FreckleToken:lx3gi6pxdjtjn57afp8c2bv1me7g89j" https://apitest.letsfreckle.com/api/user/12345678.xml
Response codes
200 OK
is returned when the user was successfully deactivated.
422 Unprocessable Entity
is returned in case the API user is not the
account owner or the account owner is trying to delete him- or herself.
Roles
Only the account owner can use this API method. The account owner can’t delete him- or herself.